Can’t Get Into Preprocessors? Try Zen Coding
A ton of discussion lately has been given to preprocessors. These incredibly useful tools make coding easier, faster and more maintainable, but they’re certainly not for everyone. Whether or not you’ve jumped on the preprocessor bandwagon, you should give a fresh look to an old favorite that helps you dramatically cut your coding time without reinventing your workflow with compilers and other complications: Zen Coding.
With Zen Coding, you can type a little and output a lot, just like with a preprocessor like Jade or Haml, only it expands instantly into the vanilla HTML that you love. For those that are new to the concept, I’ll walk you through how Zen Coding works and show you some of my favorite tricks, then end with a brief tutorial on getting Zen Coding up and running in Sublime Text 2.
Zen Coding vs. Preprocessors
Zen Coding has been around for a number of years, so several of you reading this are bound to think that this is too old school to merit a discussion in 2012, but I’m willing to bet that lots of new coders have never given it a shot.
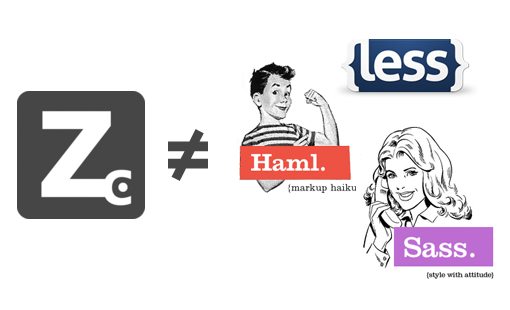
I’ll start by saying what Zen Coding isn’t: it isn’t a preprocessor in the same vein as LESS, Sass, Stylus, Jade, Haml and the like. There are similarities though. Like all of these, Zen Coding has a unique syntax that is aimed at simplifying the process of coding HTML and CSS.
However, unlike those other tools, Zen Coding doesn’t add any special features such as variables or mixins, nor does it require any extra files which have to be run through a compiler. Zen Coding outputs HTML and CSS immediately, no one has any possible way of even knowing that you used it by looking at your code.
Perhaps a closer parallel is TextExpander, an app that “expands” brief text snippets into larger ones. Basically, Zen Coding is like a giant set of prebuilt text expansion macros built specifically for HTML and CSS coders.
How Does It Work?
Enough explanation, let’s jump in and explore the actual syntax behind Zen Coding. If you’re at all familiar with HTML, CSS and the concept of the DOM, I’m sure you’ll pick it up immediately.
Let’s start with the HTML side because I think that’s where almost all of the real benefit lies. As our first basic example, let’s say we wanted to create a simple div with an ID of container, here’s how we would do it:
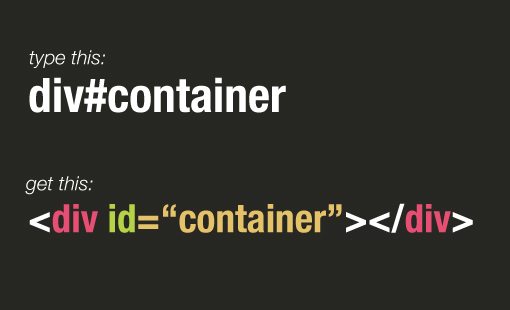
If you’re familiar with Haml, you’ll immediately see that some of the same conventions are used here. First I typed the name of the element that I wanted, in this case a div, then I used the CSS-like syntax of inserting a hashtag to indicate that I’m referring to an ID. Finally, I followed the hashtag with the actual text that I wanted assigned to the ID: “container”.
As you can see, I very nearly cut my required typing in half for this particular snippet of code. I didn’t have to bother with any starting and closing brackets or quotes, instead the structure has been stripped to a bare minimum.
We can use this knowledge as a jumping off point for other similar snippets. For instance, let’s say I want to create a paragraph with a given class. The syntax is pretty much the same, only I use a period to indicate a class (again, just like CSS).
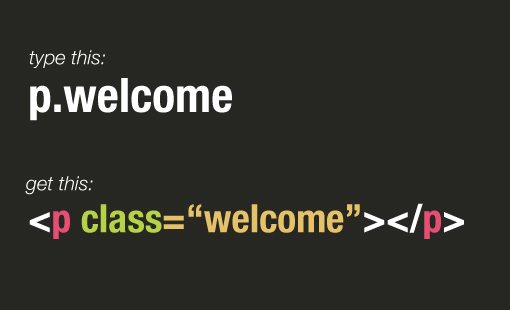
Chaining, Siblings and Children
Saving a handful of characters is quaint, but what if you’re a serious developer who can type all of that in your sleep in the span of a second? Is this Zen Coding stuff really going to be all that useful?
The answer is a resounding “yes.” In fact, the more complex your code structure becomes, the more helpful you’ll find Zen Coding to be! The real power here lies in the ability to chain together different elements.
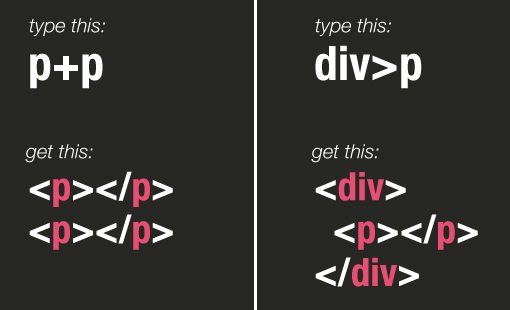
As you can see, it’s easy to create both children and siblings with the Zen Coding syntax. Sticking with the CSS theme, siblings are connected with the “+” symbol and children use the “>” symbol.
This opens up a massive amount of time and effort saving potential. Play around with this for a few minutes and your brain will quickly begin to think of the structure of HTML documents this way. Before you know it, you’ll be typing long, advanced sequences that expand into nice, fat chunks of code.
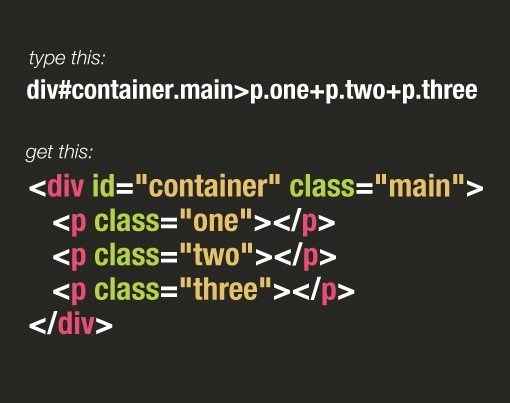
Zen CSS
Zen Coding isn’t just for HTML, it also helps you code CSS. To be honest, I don’t use this aspect of Zen Coding too much simply because most decent text editors have such good CSS auto-complete that I spend very little time manually typing out properties anyway (also, CSS auto-complete tends to compete with and override Zen Coding). The same argument could be said of HTML, but here I find Zen Coding to be much faster than the auto-complete route.
Still, this is all my personal opinion, you might find that you absolutely love the CSS side of Zen Coding. Here’s an example of how it works:
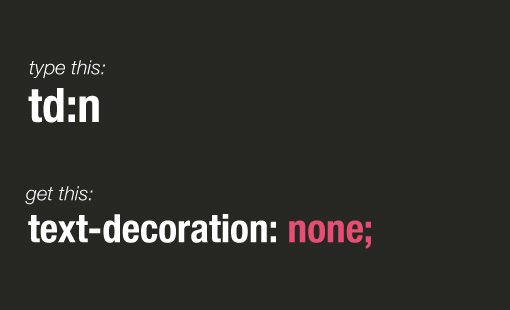
As you can see, the CSS side usually involves you typing out the initials of the property, followed by a colon and the initials of the value to assign to the property. As you might guess, there are a ton of CSS properties supported so memorization here is much trickier than with the HTML side. Be sure to check out the cheat sheets.
My Favorite HTML Macros
The Zen Coding cheat sheet can be pretty overwhelming given that there’s so much in it, so to help get you hooked right from the start, here are some of my favorite macros.
HTML5 Starter
Want to start a new HTML document with a clean slate? Simply type “html:5” and you’ll be off to a strong start.
<!--Type This--> html:5 <!--Get This--> <!DOCTYPE HTML> <html lang="en-EN"> <head> <title></title> <meta charset="UTF-8"> </head> <body> </body> </html>
br>
Stylesheet Include
Once you start that fresh HTML file, you’ll want to toss in a stylesheet. Fortunately, you can do so in a jiffy.
<!--Type This--> link:css <!--Get This--> <link rel="stylesheet" type="text/css" href="style.css" media="all">
br>
JavaScript Include
One more for the head portion of your document. After you’ve gotten the stylesheet worked out, it’s time to toss in the JavaScript.
<!--Type This--> script:src <!--Get This--> <script type="text/javascript" src=""></script>
br>
Anchor Tags
One of my first questions regarding Zen Coding was, “how do links work?” Haml is actually pretty awkward when it comes to any sort of inline elements and attributes so I was worried that this might be the same.
However, instead of coming up with a complicated scheme for inserting the link text and URL, you simply type “a” and the core structure of the anchor is created and made ready for you to fill in. It could probably be a little better, but at least it’s easy to remember!
<!--Type This--> a <!--Get This--> <a href=""></a> <!--Type This--> a:link <!--Get This--> <a href="http://"></a>
br>
Multiply Elements
Zen Coding has a handy multiplier trick that makes it easy to create a certain number of a specific elements. Once again, the process is very intuitive, just use the “*” symbol and the number of the element that you’d like to create.
<!--Type This--> p*3 <!--Get This--> <p></p> <p></p> <p></p> <!--Type This--> ul>li.someclass*4 <!--Get This--> <ul> <li class="someclass"></li> <li class="someclass"></li> <li class="someclass"></li> <li class="someclass"></li> </ul>
br>
Increment Number
Another really cool trick that you can pull off while multiplying elements is to include an incrementing number in the class or ID value. To do this, you implement the “$” symbol.
<!--Type This--> ul>li.someclass-$*5 <!--Get This--> <ul> <li class="someclass-1"></li> <li class="someclass-2"></li> <li class="someclass-3"></li> <li class="someclass-4"></li> <li class="someclass-5"></li> </ul>
br>
How Do I Make This Work?
Zen Coding is supported in tons of different text editors and is occasionally even built in, as is the case with Espresso. Be sure to check the Zen Coding Google Project Page to download the plugins for your editor.
How To Install Zen Coding on Sublime Text 2
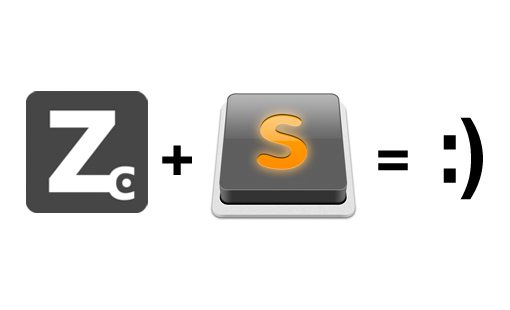
I’ve definitely been bitten by the Sublime Text bug. I simply love this text editor and can’t get enough of its extensibility and flexibility. One quick and easy way to make Sublime Text 2 even better is to add Zen Coding support.
To do this, you’ll need to grab the free Sublime Package Control download from Will Bond. This is an awesome package manager that makes finding and installing Sublime text plugins incredibly easy.
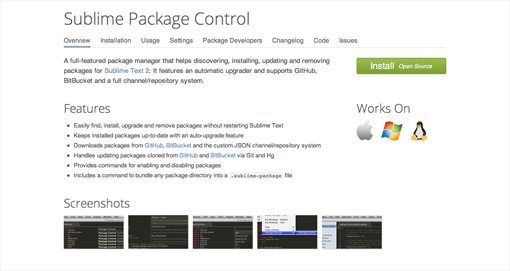
Once you’ve got that installed, hit Command+Shift+P (Command = Control for PC folks) to bring up the Command Palette and type the word “install”. You should see an item that says “Package Control: Install Package”, hit enter on that option.
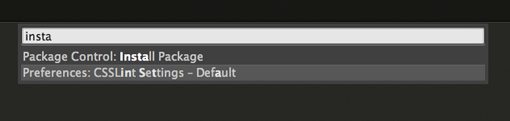
From here, type “Zen Coding” and you should see the package. Simply hit enter again and the package will be installed (restart if it doesn’t take effect). Start a new HTML document, type out an abbreviation, hit tab, and watch the magic (make sure your syntax is set to HTML or it won’t work).
Sublime text is already a fantastic editor and using it conjunction with Zen Coding just makes me smile. It’s a killer combination that you won’t want to miss out on.
Do You Use Zen Coding?
To be honest, I really missed the boat on Zen Coding when it was popular a couple of years ago. I always found the Espresso integration to be a little clunky and since that was my editor of choice, I never explored it further. Now that I’m on Sublime Text I just love how quickly I can use Zen Coding to bust out a complex HTML structure without the hassle of tags, returns and the like.
What do you think? Do you use Zen Coding for your HTML? Do you prefer it over using a preprocessor to do something similar? How about with your CSS? Do you think Zen Coding is useful for typing CSS or are you fine with auto-complete?